If you’re a web developer like me, you probably use console a lot. We use it to debug JavaScript code, to print out the contents of an object etc.
I use built-in Chrome tools, which is enough for most of the cases. I also use a Vue Devtools extension, when I build Vue.js apps, and I love it.
Here is a short and sweet tip on how to print JavaScript object using console.log()
.
How to print out the content of the JavaScript object
Suppose you have a JavaScript object:
var person = {
name: 'Nedd',
surname: 'Stark',
child: {
child1: 'child1Value'
}
}
To print out the content of person, you first need to stringify it using JSON.stringify()
method.
str = JSON.stringify(person, null, 2); // it will also indent the output
Then pass it to console.log()
console.log(str);
Your console output will be
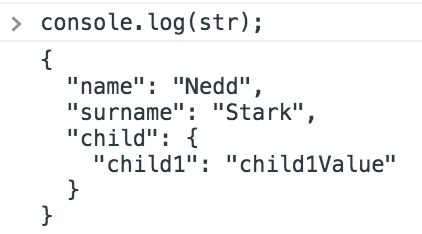
If you need to reverse the operation use JSON.parse()
method
person = JSON.parse(str);
Bonus console tips
#1 console.clear()
When your console log becomes too long you can clear its content using console.clear()
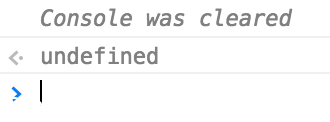
#2 console.count()
If you want to count how many times your function has been called, you can use the console.count()
function. It also takes an optional parameter “label”. Here is an example:
let user = "";
function greet() {
console.count(user);
return "hi " + user;
}
user = "bob";
greet();
user = "alice";
greet();
greet();
console.count("alice");
You’ll see output like this:
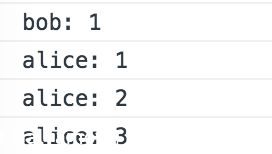
#3 console.table()
This one is my favourite, you can print a proper table with the objects using console.table()
function.
var people = [
{ name: "Nedd", surname: "Stark" },
{ name: "Robb", surname: "Stark" }
]
console.table(people)
You’ll see this beautiful table inside the console.

If you find this post useful, please let me know in the comments below.
Cheers,
Renat Galyamov
PS: Make sure you check other posts e.g. how to check if a user has scrolled to the bottom in Vue.js or how to do Vue.js polling using setInterval().
Want to share this with your friends?
👉renatello.com/javascript-print-object
Incoming search terms:
console.log() can show the objects. not need JSON.stringify